I want to share a little snippet in case anyone is interested in writing video files from cpp context using FFMPEG, I’ve made it for usage with OpenFrameworks.
It’s basically a simple wrapper around the encoding mechanism of FFMPEG, so you can easily just say which file to write to and start dumping images into it. It will take care of choosing the codec, writing headers and trailers, encode (compress) the frames and write them to the file.
Author: Roy
I wanna share some code for 2D curve tracking with a particle filter, implementing the body of work of Tony Heap and David Hogg. These guys presented a relatively easy to implement method for tracking deformable curves through space and change in form using a Hierarchical Point Distribution Models (HPDM), which is another elegant way to store shape priors. Granted, it is not perfect, but for a simple 2D shape like a hand it works pretty good, and rather fast as well.
Let’s dive in then,
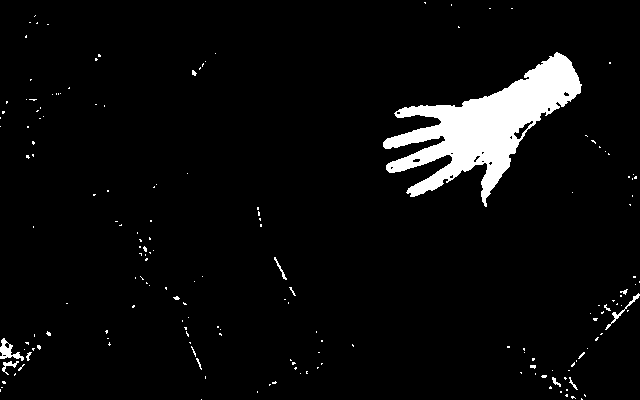
Sharing a bit of code I created for skin detection.
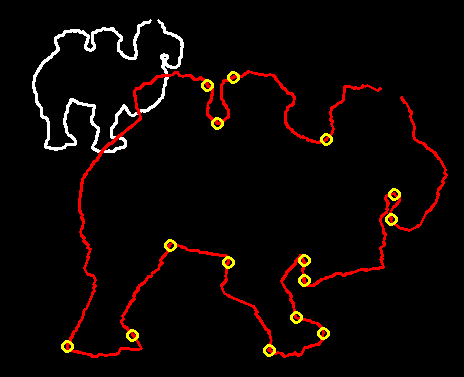
Hello,
I wanna share some code I’ve been working on lately that implements smooth shape manipulation using Moving Least Squares. More specifically, the excellent simple and powerful method by Schaefer et al. from Texas A&M and Rice universities (great paper). The method was written for image deformation but very straightforward modifications made it work perfectly for 2D shapes and open curves.
Let’s get down to business
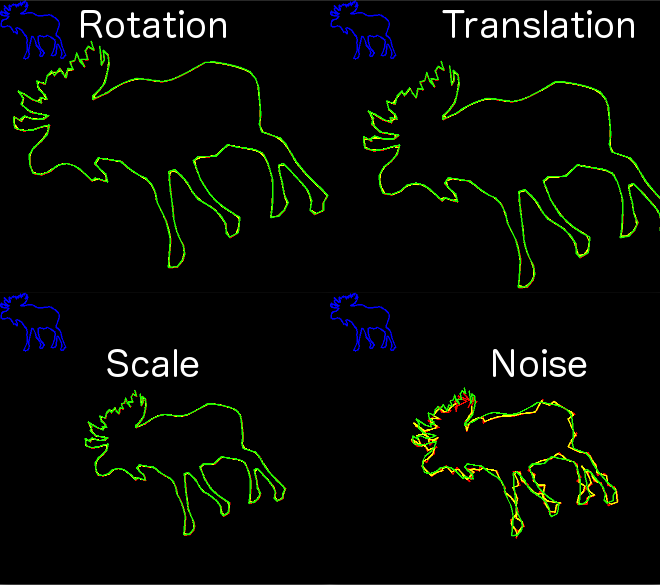
Just sharing some code and ideas for matching 2D curves. I was working for a while on matching 2D curves to discover shapes in images, but it didn’t work out, what did succeed is this 2D curve matcher that seems to be very robust for certain applications. It’s based on ideas from the Heat Kernel Signature and the CSS Image (that I introduced in my latest post), all around inspecting curves under different level of smoothing.
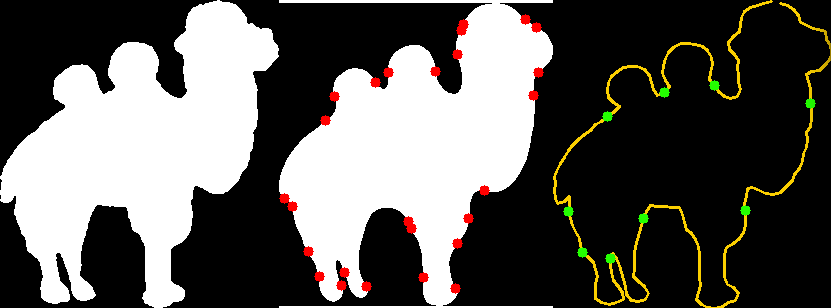
I’m so glad to be back to work on a graphics project (of which you will probably hear later), because it takes me back to reading papers and implementing work by talented people. I want share a little bit of utilities I’ve developed for working with 2D curves in OpenCV.
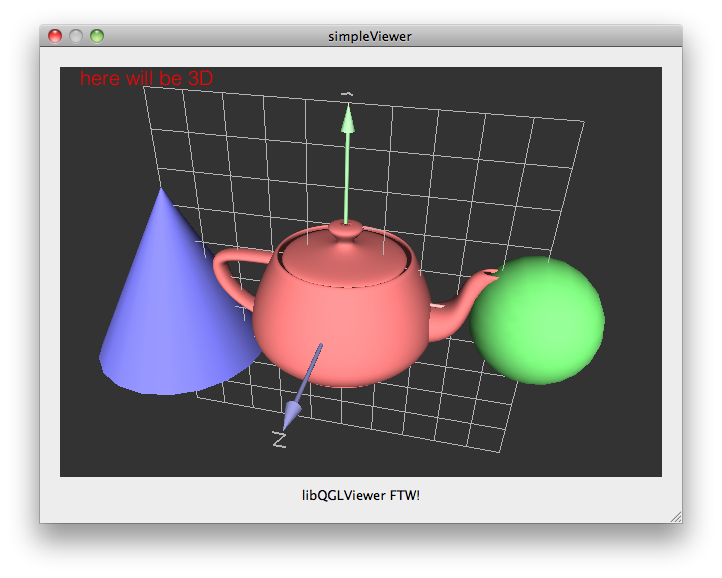
While looking for a very simple way to start up an OpenGL visualizer for quick 3D hacks, I discovered an excellent library called libQGLViewer, and I want to quickly show how easy it is to setup a 3D environment with it. This library provides an easy to access and feature-rich QtWidget you can embed in your UIs or use stand-alone (this may sound like a marketing thing, but they are not paying me anything 🙂
This is based on the library’s own examples at: http://www.libqglviewer.com/examples/index.html, and some of the examples that come with the library source itself.
Let’s see how it’s done

So I was contacted earlier by someone asking about the Head Pose Estimation work I put up a while back. And I remembered that I needed to go back to that work and fix some things, so it was a great opportunity.
I ended up making it a bit nicer, and it’s also a good chance for us to review some OpenCV-OpenGL interoperation stuff. Things like getting a projection matrix in OpenCV and translating it to an OpenGL ModelView matrix, are very handy.
Let’s get down to the code.
Just a snippet of code if someone out there needs it. I keep coming back to it, so I thought why not share it…
#include <iostream> #ifndef WIN32 #include <dirent.h> #endif using namespace std; void open_imgs_dir(char* dir_name, std::vector<std::string>& file_names) { if (dir_name == NULL) { return; } string dir_name_ = string(dir_name); vector<string> files_; #ifndef WIN32 //open a directory the POSIX way DIR *dp; struct dirent *ep; dp = opendir (dir_name); if (dp != NULL) { while (ep = readdir (dp)) { if (ep->d_name[0] != '.') files_.push_back(ep->d_name); } (void) closedir (dp); } else { cerr << ("Couldn't open the directory"); return; } #else //open a directory the WIN32 way HANDLE hFind = INVALID_HANDLE_VALUE; WIN32_FIND_DATA fdata; if(dir_name_[dir_name_.size()-1] == '\\' || dir_name_[dir_name_.size()-1] == '/') { dir_name_ = dir_name_.substr(0,dir_name_.size()-1); } hFind = FindFirstFile(string(dir_name_).append("\\*").c_str(), &fdata); if (hFind != INVALID_HANDLE_VALUE) { do { if (strcmp(fdata.cFileName, ".") != 0 && strcmp(fdata.cFileName, "..") != 0) { if (fdata.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) { continue; // a diretory } else { files_.push_back(fdata.cFileName); } } } while (FindNextFile(hFind, &fdata) != 0); } else { cerr << "can't open directory\n"; return; } if (GetLastError() != ERROR_NO_MORE_FILES) { FindClose(hFind); cerr << "some other error with opening directory: " << GetLastError() << endl; return; } FindClose(hFind); hFind = INVALID_HANDLE_VALUE; #endif file_names.clear(); file_names = files_; }
Enjoy
Roy.
Hello
Sorry for the bombardment of posts, but I want to share some stuff I’ve been working on lately, so when I find time I just shoot the posts out.
So this time I’ll talk shortly about how to get an estimation of a rigid transformation between two clouds, that potentially are also of different scale. You will end up with a rigid transformation (Rotation Translation) and a scale factor, son in fact it will be a Similarity Transformation. We will first find the right scale, and then find the right transformation, given there is one (but we will find the best transformation there is).